03 JavaScript: Password Generator
This week’s homework requires you to modify starter code to create an application that enables employees to generate random passwords based on criteria that they’ve selected. This app will run in the browser and will feature dynamically updated HTML and CSS powered by JavaScript code that you write. It will have a clean and polished, responsive user interface that adapts to multiple screen sizes.
The password can include special characters. If you’re unfamiliar with these, see this list of password special characters from the OWASP Foundation.

Acceptance Criteria
The following image shows the web application’s appearance and functionality:
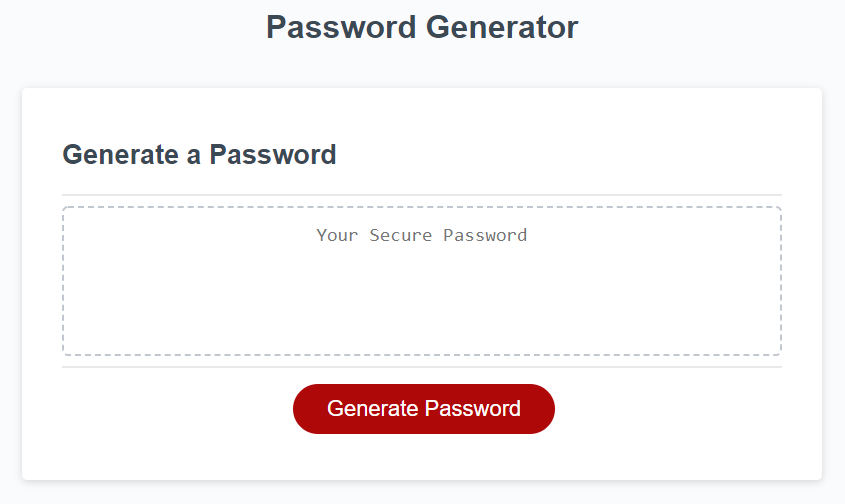
Grading Requirements
This homework is graded based on the following criteria:
Technical Acceptance Criteria: 40%
Satisfies all of the preceding acceptance criteria plus the following:
- The homework should not produce any errors in the console when you inspect it using Chrome DevTools.
Deployment: 32%
Application deployed at live URL.
Application loads with no errors.
Application GitHub URL submitted.
GitHub repository that contains application code.
Application Quality: 15%
Application user experience is intuitive and easy to navigate.
Application user interface style is clean and polished.
Application resembles the mock-up functionality provided in the homework instructions.
Repository Quality: 13%
Repository has a unique name.
Repository follows best practices for file structure and naming conventions.
Repository follows best practices for class/id naming conventions, indentation, quality comments, etc.
Repository contains multiple descriptive commit messages.
Repository contains quality readme file with description, screenshot, and link to deployed application.
You are required to submit the following for review:
The URL of the deployed application.
The URL of the GitHub repository, with a unique name and a readme describing the project.
© 2021 Trilogy Education Services, LLC, a 2U, Inc. brand. Confidential and Proprietary. All Rights Reserved.
03 JavaScript: Password Generator
Create an application that generates a random password based on user-selected criteria. This app will run in the browser and feature dynamically updated HTML and CSS powered by your JavaScript code. It will also feature a clean and polished user interface and be responsive, ensuring that it adapts to multiple screen sizes.
If you are unfamiliar with special characters, take a look at some examples .
Acceptance Criteria
The following image demonstrates the application functionality:

password generator demo
You are required to submit the following for review:
The URL of the deployed application.
The URL of the GitHub repository. Give the repository a unique name and include a README describing the project.
© 2019 Trilogy Education Services, a 2U, Inc. brand. All Rights Reserved.
Refactoring-HW-2
03 javascript: password generator.
This Homework assignment we were tasked with adding javascript to already exisitng code to create a functioning random password generator with options for Capitalization , Lowercasing , numbers and special characters.
Acceptance Criteria
this is a link to the webpage
https://jcxxz.github.io/Refactoring-HW-2/

challenge03-javascript
Javascript challenge: password generator, description.
For this challenge, the goal was to create an application that generates a random password based on criteria from user input.
This browser application will help users create a secure password that is as complex as needed to fit their desired criteria.
Table of Contents
Once a user enters the web application, they will be able to click the main red button in the middle of the page marked “Generate Password”. Once clicked, multiple alerts will prompt a series of questions determining their desired criteria for their password. The user will be able to chose the following criteria: length, lowercase, uppercase, numeric, and special characters. The application only requires the user to have a length between 8-128 chacters and for at least one chacter type to be selected. All other criteria are optional. Once all the prompts have been correctly answered, a password is generated that matches the selected criteria and is displaed on the page.
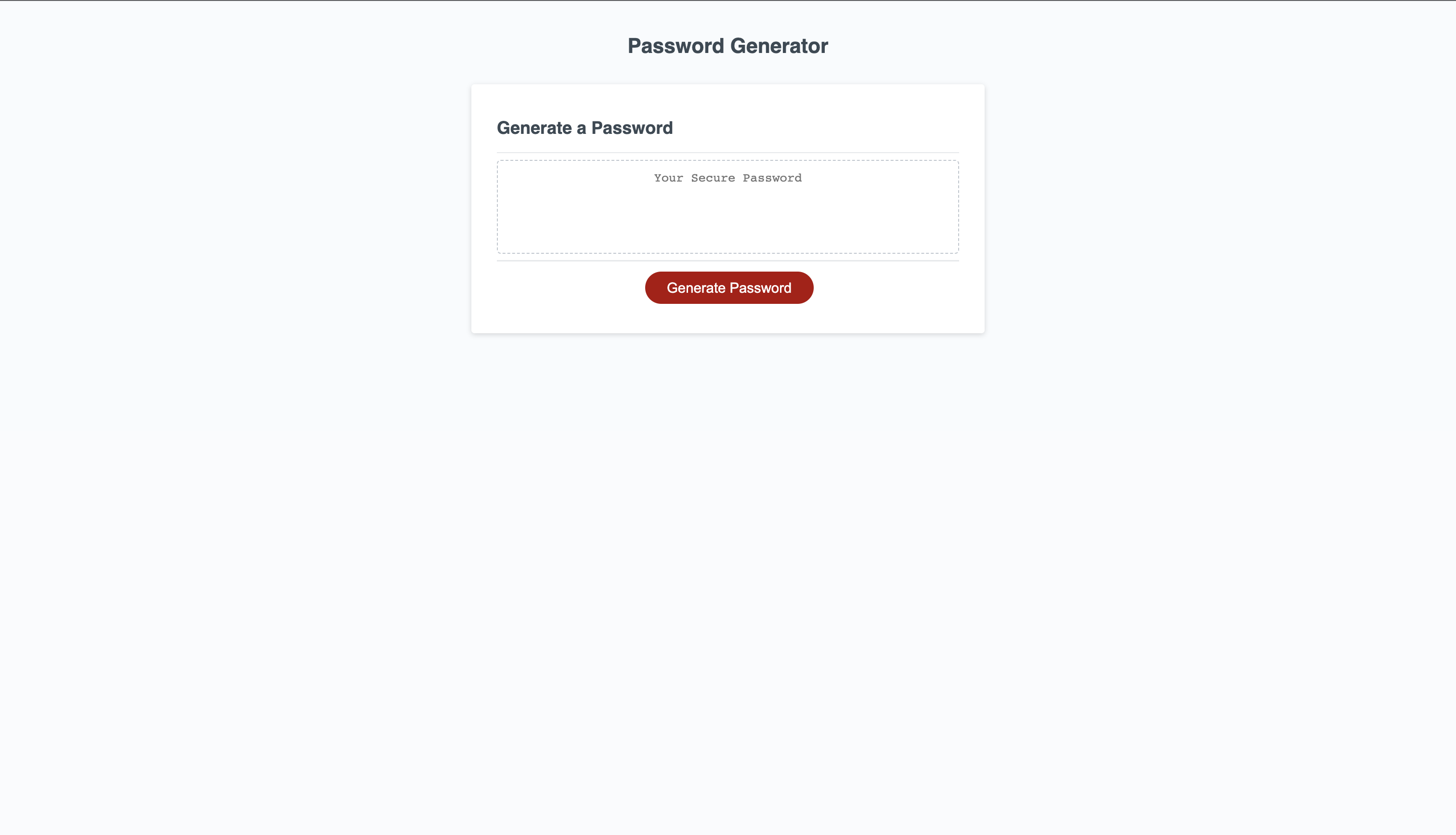
This application was made possible with the help of starter code provided by edX @ GeorgiaTech Coding BootCamp, whose GitHub repository page is linked below: https://github.com/coding-boot-camp/friendly-parakeet
GitHub Page: https://github.com/esanchez8k/challenge03-javascript#screenshot
Deployed Application: https://esanchez8k.github.io/challenge03-javascript/
js_password_generator
Week 3 javascript homework challenge
The purpose of this website is to allow the user to the use to generate a random password with a specific chosen number of characters (between 8 - 128 characters). The random password is then written on the page.
Deployement link: https://slingshort.github.io/js_password_generator/
The user will have the choice of whether or not to include: - Special characters - Lower case characters - Upper case characters - Numerical characters
Features: - All boolean values of window.confirm questions are logged in console for easy checking - If response is invalid, this is also console logged. An invalid response also prompts a window alert with more specific instructions
Things that can be improved: - The extensive if/else statements could potentially be trimmed down with more resourceful use of logical comparison operators
The following image demonstrates the functionality of the page with a view of the console:

Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
This is an application that generates a random password based on user-selected criteria. This app will run in the browser and feature dynamically updated HTML and CSS powered by my JavaScript code.
wailam706/Unit-03-JavaScript-Homework-Password-Generator
Folders and files, repository files navigation, unit-03-javascript-homework-password-generator.
Production page: https://dhens.github.io/Unit-03-JavaScript-Homework-Password-Generator/
This repo contains:
- This README
- A javascript-powered password generator page that uses user-selected criteria for what the passwords characters are
- Bootstrap 4 and my own stylesheet
Known Issues
- Passwords generated are not entirely random as each character type is chosen sequentially
What I Learned:
- Bootstrap allows you to focus on the functionality of the page, rather than spending a lot of time just making the website look the way it needs to.
- Knowing Javascript and HOW to use it in it's full extent is extremely valuble and surface-level knowledge never holds up on more complex projects, such as this.
Conclusion:
- I needs to study Javascript more so that I know how to use the built in features and methods so that I can confidently create powerful and dyamic webpages.
- JavaScript 52.3%
Password Generator
Generate a password.

IMAGES
VIDEO
COMMENTS
This application is a password generator powered by Javascript. The application can take between 8 to 128 characters, though it does accept down to 1. The application does the following for password generation; Clipboard for copying the password to the user's clipboard.
Create an application that generates a random password based on user-selected criteria. This app will run in the browser and feature dynamically updated HTML and CSS powered by your JavaScript code. \n. The user will be prompted to choose from the following password criteria: \n \n \n. Length (must be between 8 and 128 characters) \n \n \n ...
Unit 03 JavaScript Homework: Password Generator. Contribute to Emi-dev/HW3-Password-Generator development by creating an account on GitHub.
This week’s homework requires you to modify starter code to create an application that enables employees to generate random passwords based on criteria that they’ve selected. This app will run in the browser and will feature dynamically updated HTML and CSS powered by JavaScript code that you write.
03 JavaScript: Password Generator. Create an application that generates a random password based on user-selected criteria. This app will run in the browser and feature dynamically updated HTML and CSS powered by your JavaScript code.
This Homework assignment we were tasked with adding javascript to already exisitng code to create a functioning random password generator with options for Capitalization , Lowercasing , numbers and special characters.
For this challenge, the goal was to create an application that generates a random password based on criteria from user input. This browser application will help users create a secure password that is as complex as needed to fit their desired criteria.
js_password_generator. Week 3 javascript homework challenge. The purpose of this website is to allow the user to the use to generate a random password with a specific chosen number of characters (between 8 - 128 characters). The random password is then written on the page.
This is an application that generates a random password based on user-selected criteria. This app will run in the browser and feature dynamically updated HTML and CSS powered by my JavaScript code....
Welcome! Click the button below to begin generating your secure password.